Remixful
Active Member
Right now, I'm starting to delve into game developing. I've learned a lot of C#, I think at least the basics to at least get started on working on something, instead of text based games in a Console Application. I attempted Unity, but I just didn't feel comfortable, because I wanted to do 2D more than 3D. So I choose to finally go with Monogame, and work on Visual Studio... where I feel like home. Currently, I'm reading and watching some tutorials on XNA, since there are much more tutorials on XNA then there are on Monogame. (The amount of tutorials on Monogame are actually really low.)
But no worries, if I learn XNA, I can use the same exact knowledge to use in Monogame (since Monogame implements XNA).
I haven't done much yet, but reading, and doing a little experimenting on the side. One thing I'm trying hard to work on is my "spaghetti code", and logic.
As you can see, I did add boundaries to where the character can walk. Also if I hold shift the character can run. I've also done sprite animation, as you can see.
So far I haven't learnt collisions, I believe it's Rectangle collisions, but I'm only on the 3rd chapter of my book. XD
Anyways.. this is the first step.. my first big project is going to be to recreate a Pokemon game.
(Of course, I'm only going to do little steps of experimenting on the way for now though, and make small little games too.)
Wish me luck!
But no worries, if I learn XNA, I can use the same exact knowledge to use in Monogame (since Monogame implements XNA).
I haven't done much yet, but reading, and doing a little experimenting on the side. One thing I'm trying hard to work on is my "spaghetti code", and logic.
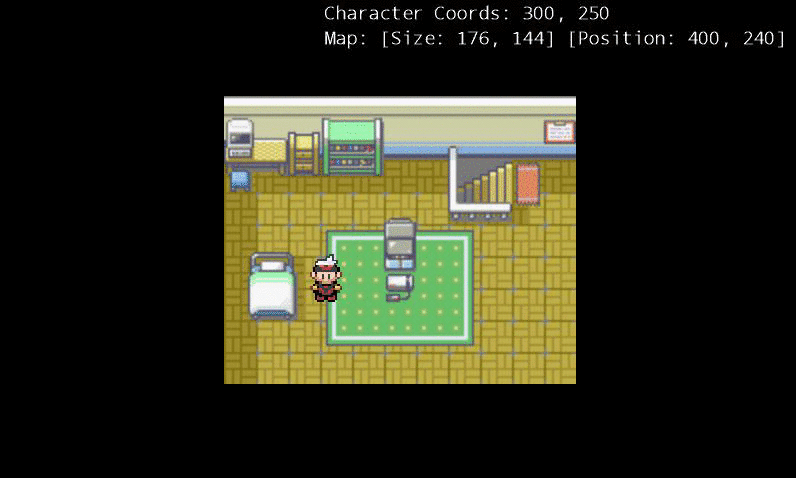
As you can see, I did add boundaries to where the character can walk. Also if I hold shift the character can run. I've also done sprite animation, as you can see.
So far I haven't learnt collisions, I believe it's Rectangle collisions, but I'm only on the 3rd chapter of my book. XD
Anyways.. this is the first step.. my first big project is going to be to recreate a Pokemon game.
(Of course, I'm only going to do little steps of experimenting on the way for now though, and make small little games too.)
Wish me luck!